Hoy vamos a trabajar en un caso práctico algo más complejo(pero tampoco tan dificil) que reúne los principales puntos impartidos en el curso de CrewAI en Deeplearning. Con este sistema de agentes podremos rastrear las skills demandadas en las ofertas de trabajo para ese puesto que quieres alcanzar, modifica tu Curriculum para aplicar y adicionalmente te prepara para la entrevista!
Lo que tienes en este articulo es oro en polvo y una gran práctica para trabar con Agentes de Inteligencia artificial haciendo uso de muchas de las posibilidades del framework de CrewAI. Si no tienes claro los conceptos básicos sobre agentes de IA te invito a leer esta Introducción a los agentes de AI con el framework de CrewAI
Cuál es el objetivo de esta demostración:
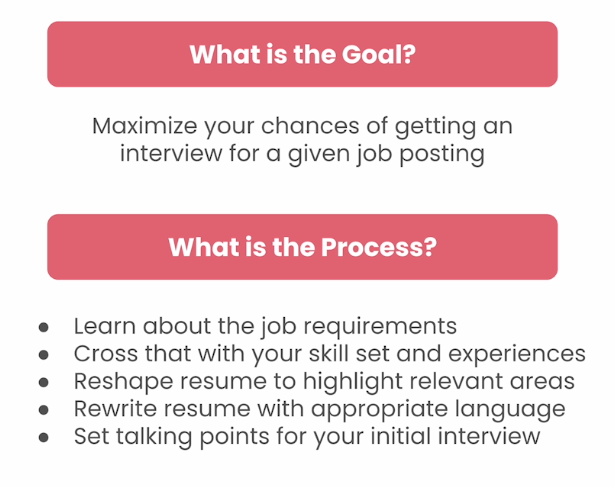
¡Vamos al lio!
Lo primero como siempre, las dependencias necesarias:
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Task, Crew
Llamamos a la API de openai, en este ejemplo utilizamos GTP 4 turbo por su gran capacidad de respuesta y entendimiento del contexto actualizado.
import os
from utils import get_openai_api_key, get_serper_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
os.environ["SERPER_API_KEY"] = get_serper_api_key()
CrewAI Tools
Utilizamos 4 herramientas del framework que nos permiten:
- Revisar resultados de búsqueda en la SERP(con la API de SERPER, gratuita para comenzar)
- Scrapear los resultados de búsqueda
- Leer el archivo de nuestro CV
- Realizar una búsqueda semántica RAG sobre nuestro CV
from crewai_tools import (
FileReadTool,
ScrapeWebsiteTool,
MDXSearchTool,
SerperDevTool
)
search_tool = SerperDevTool()
scrape_tool = ScrapeWebsiteTool()
read_resume = FileReadTool(file_path='./cv_seo.md')
semantic_search_resume = MDXSearchTool(mdx='./cv_seo.md')
Creating Agents
Creamos 4 agentes para que trabajen en equipo en este proyecto
# Agent 1: Researcher
researcher = Agent(
role="SEO Job Researcher",
goal="Make sure to do amazing analysis on "
"job posting to help job applicants",
tools = [scrape_tool, search_tool],
verbose=True,
backstory=(
"As a Job Researcher, your prowess in "
"navigating and extracting critical "
"information from job postings is unmatched."
"Your skills help pinpoint the necessary "
"qualifications and skills sought "
"by employers, forming the foundation for "
"effective application tailoring."
)
)
# Agent 2: Profiler
profiler = Agent(
role="Personal Profiler for SEO experts",
goal="Do increditble research on job applicants "
"to help them stand out in the job market",
tools = [scrape_tool, search_tool,
read_resume, semantic_search_resume],
verbose=True,
backstory=(
"Equipped with analytical prowess, you dissect "
"and synthesize information "
"from diverse sources to craft comprehensive "
"personal and professional profiles, laying the "
"groundwork for personalized resume enhancements."
)
)
# Agent 3: Resume Strategist
resume_strategist = Agent(
role="Resume Strategist for SEO experts",
goal="Find all the best ways to make a "
"resume stand out in the job market.",
tools = [scrape_tool, search_tool,
read_resume, semantic_search_resume],
verbose=True,
backstory=(
"With a strategic mind and an eye for detail, you "
"excel at refining resumes to highlight the most "
"relevant skills and experiences, ensuring they "
"resonate perfectly with the job's requirements."
)
)
# Agent 4: Interview Preparer
interview_preparer = Agent(
role="SEO experts Interview Preparer",
goal="Create interview questions and talking points "
"based on the resume and job requirements",
tools = [scrape_tool, search_tool,
read_resume, semantic_search_resume],
verbose=True,
backstory=(
"Your role is crucial in anticipating the dynamics of "
"interviews. With your ability to formulate key questions "
"and talking points, you prepare candidates for success, "
"ensuring they can confidently address all aspects of the "
"job they are applying for."
)
)
Creating Tasks
Creamos las tareas para cada uno de nuestros agentes
# Task for Researcher Agent: Extract Job Requirements
research_task = Task(
description=(
"Analyze the job posting URL provided ({job_posting_url}) "
"to extract key skills, experiences, and qualifications "
"required. Use the tools to gather content and identify "
"and categorize the requirements."
),
expected_output=(
"A structured list of job requirements, including necessary "
"skills, qualifications, and experiences."
),
agent=researcher,
async_execution=True
)
# Task for Profiler Agent: Compile Comprehensive Profile
profile_task = Task(
description=(
"Compile a detailed personal and professional profile "
"using my Linkedin profile ({linkedin_profile_url}) URLs, and personal write-up "
"({personal_writeup}). Utilize tools to extract and "
"synthesize information from these sources."
),
expected_output=(
"A comprehensive profile document that includes skills, "
"project experiences, contributions, interests, and "
"communication style."
),
agent=profiler,
async_execution=True
)
#You can pass a list of tasks as context to a task.
#The task then takes into account the output of those tasks in its execution.
#The task will not run until it has the output(s) from those tasks
# Task for Resume Strategist Agent: Align Resume with Job Requirements
resume_strategy_task = Task(
description=(
"Using the profile and job requirements obtained from "
"previous tasks, tailor the resume to highlight the most "
"relevant areas. Employ tools to adjust and enhance the "
"resume content. Make sure this is the best resume even but "
"don't make up any information. Update every section, "
"inlcuding the initial summary, work experience, skills, "
"and education. All to better reflrect the candidates "
"abilities and how it matches the job posting."
),
expected_output=(
"An updated resume that effectively highlights the candidate's "
"qualifications and experiences relevant to the job."
),
output_file="tailored_resume.md",
context=[research_task, profile_task],
agent=resume_strategist
)
# Task for Interview Preparer Agent: Develop Interview Materials
interview_preparation_task = Task(
description=(
"Create a set of potential interview questions and talking "
"points based on the tailored resume and job requirements. "
"Utilize tools to generate relevant questions and discussion "
"points. Make sure to use these question and talking points to "
"help the candiadte highlight the main points of the resume "
"and how it matches the job posting."
),
expected_output=(
"A document containing key questions and talking points "
"that the candidate should prepare for the initial interview."
),
output_file="interview_materials.md",
context=[research_task, profile_task, resume_strategy_task],
agent=interview_preparer
)
Creating the Crew
job_application_crew = Crew(
agents=[researcher,
profiler,
resume_strategist,
interview_preparer],
tasks=[research_task,
profile_task,
resume_strategy_task,
interview_preparation_task],
verbose=True
)
Running the Crew
job_application_inputs = {
'job_posting_url': 'https://www.linkedin.com/jobs/view/3952546138/?refId=7c1d62a0-682e-3643-8db3-f0549f53f5d2',
'linkedin_profile_url': 'https://www.linkedin.com/in/gabinoguera/',
'personal_writeup': """Hi, I am Gabriel, currently IT Project Manager at Educa Edtech Group of the
Euroinnova Group. My main career has been linked to digital marketing and SEO but
in the last few years I have specialized in artificial intelligence and programming,
mainly with Python.
I am currently finishing a Master in artificial intelligence and knowledge engineering
that includes knowledge and management of data mining and visualization tools
such as Hadoop or Power BI, among others.
Additionally I have developed AI pipelines using Langchain, GPT, Tensorflow, NLP,
Hugging Face, etc. mainly for applications related to content generation and
analytics of trends, traffic, audience and end-user understanding to improve
recommendation systems, advertising and personalization.
I have worked in both fast-paced and multitask startup environments as well as in
larger companies like the current one, where there are more solid and specialized
teamwork structures with agile methodologies"""
}
¡A correr!
### this execution will take a few minutes to run
result = job_application_crew.kickoff(inputs=job_application_inputs)
Visualizamos nuestro nuevo CV
#Dislplay the generated tailored_resume.md file.
from IPython.display import Markdown, display
display(Markdown("./tailored_resume.md"))
Visualizamos los key points para nuestra entrevista
# Dislplay the generated interview_materials.md file.
display(Markdown("./interview_materials.md"))
Resultados
La verdad que los resultados me han dejado sorprendido. Si bien no se hace uso del total de información que podría contener en mi CV si que se logra un enfoque más preciso sobre la descripción de las skills y experiencias relacionadas directamente con el puesto de trabajo ofertado.
Adicionalmente los keypoints para la entrevista también son muy utiles para mentalizarse sobre las posibles preguntas que pueden caer, ya que se estructuran de manera similar a los requerimientos de la oferta de trabajo.
Cabe aclarar que en el curso impartido por CrewAI se utiliza otra url y un repositoriod e Github, en este caso la he adaptado a Linkedin, pasando una oferta de trabajo en esta red social y mi profile personal.
Espero que puedan experimetnar y conseguir su nuevo puesto de trabajo con la ayuda de estos agentes, incluso se me ocurren unas cuantas ideas para mejorar este pipeline, las tuyas son bienvenidas en los comentarios.